HOME | DD
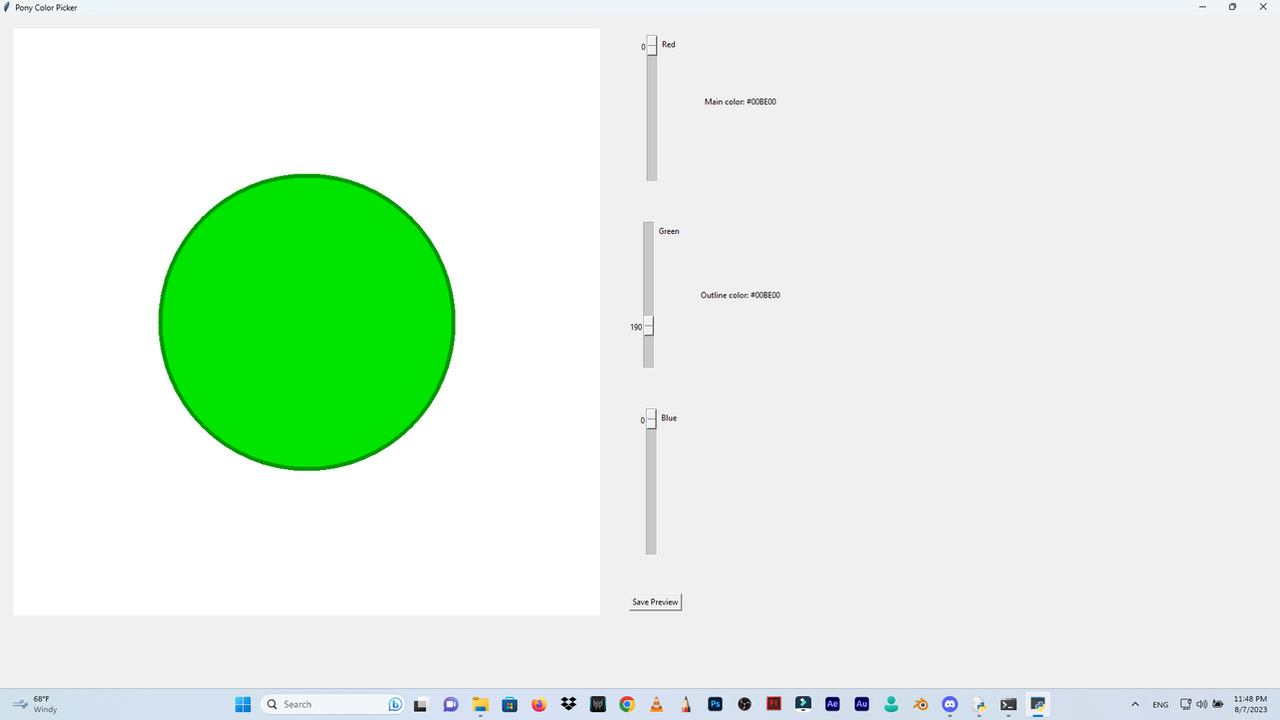
Published: 2023-08-08 06:52:12 +0000 UTC; Views: 287; Favourites: 0; Downloads: 0
Redirect to original
Description
i been working Python script that makes all of this possible.Here is the code:
import tkinter as tk
from tkinter import filedialog
from PIL import Image, ImageDraw
def adjust_brightness(hex_color, factor):
r = int(hex_color[1:3], 16)
g = int(hex_color[3:5], 16)
b = int(hex_color[5:7], 16)
r = min(255, max(0, int(r * factor)))
g = min(255, max(0, int(g * factor)))
b = min(255, max(0, int(b * factor)))
return "#{:02X}{:02X}{:02X}".format(r, g, b)
def update_color(event=None):
r = red_slider.get()
g = green_slider.get()
b = blue_slider.get()
color_hex = "#{:02X}{:02X}{:02X}".format(r, g, b)
body_color = adjust_brightness(color_hex, 1.2)
outline_color = adjust_brightness(color_hex, 0.8)
body_canvas.itemconfig(body_ball, fill=body_color, outline=outline_color)
main_color_label.config(text=f"Main color: {color_hex}")
outline_color_label.config(text=f"Outline color: {color_hex}")
global current_color
current_color = color_hex
def save_preview_as_image():
if current_color:
file_path = filedialog.asksaveasfilename(defaultextension=".png", filetypes=[("PNG files", "*.png"), ("JPEG files", "*.jpg")])
if file_path:
# Create a blank image
img = Image.new("RGB", (200, 200), "white")
draw = ImageDraw.Draw(img)
# Draw the body and outline
body_color = adjust_brightness(current_color, 1.2)
outline_color = adjust_brightness(current_color, 0.8)
draw.ellipse((40, 40, 160, 160), fill=body_color, outline=outline_color, width=6)
# Save the image
img.save(file_path)
print("Image saved successfully!")
# Create the main window
root = tk.Tk()
root.title("Pony Color Picker")
# Set the window size to fit a 1920x1080 screen resolution
window_width = 1920
window_height = 1080
root.geometry(f"{window_width}x{window_height}")
# Make the window transparent
root.attributes("-alpha", 100) # You can adjust the transparency level
# Calculate widget sizes based on the window size
canvas_size = min(window_width, window_height) - 200
slider_size = int(canvas_size / 4)
# Create canvas for the pony
body_canvas = tk.Canvas(root, width=canvas_size, height=canvas_size, bg="white", highlightthickness=0)
# Create circular ball for body
ball_radius = int(canvas_size / 4)
body_ball = body_canvas.create_oval(ball_radius, ball_radius, canvas_size - ball_radius, canvas_size - ball_radius, fill="#000000", outline="#000000", width=6) # Increased outline width
# Create sliders for red, green, and blue
red_slider = tk.Scale(root, from_=0, to=255, orient="vertical", label="Red", length=slider_size, command=update_color)
green_slider = tk.Scale(root, from_=0, to=255, orient="vertical", label="Green", length=slider_size, command=update_color)
blue_slider = tk.Scale(root, from_=0, to=255, orient="vertical", label="Blue", length=slider_size, command=update_color)
# Create labels for main color and outline color
main_color_label = tk.Label(root, text="Main color: #000000")
outline_color_label = tk.Label(root, text="Outline color: #000000")
# Create a button to save the preview as an image
save_button = tk.Button(root, text="Save Preview", command=save_preview_as_image)
# Layout the widgets
body_canvas.grid(row=0, column=0, padx=20, pady=20, rowspan=4)
red_slider.grid(row=0, column=1, padx=20, pady=20)
green_slider.grid(row=1, column=1, padx=20, pady=20)
blue_slider.grid(row=2, column=1, padx=20, pady=20)
main_color_label.grid(row=0, column=2, columnspan=2, pady
outline_color_label.grid(row=1, column=2, columnspan=2)
save_button.grid(row=3, column=1, pady=20)
# Initialize the color preview
current_color = "#000000"
update_color()
# Start the Tkinter main loop
root.mainloop()