HOME | DD
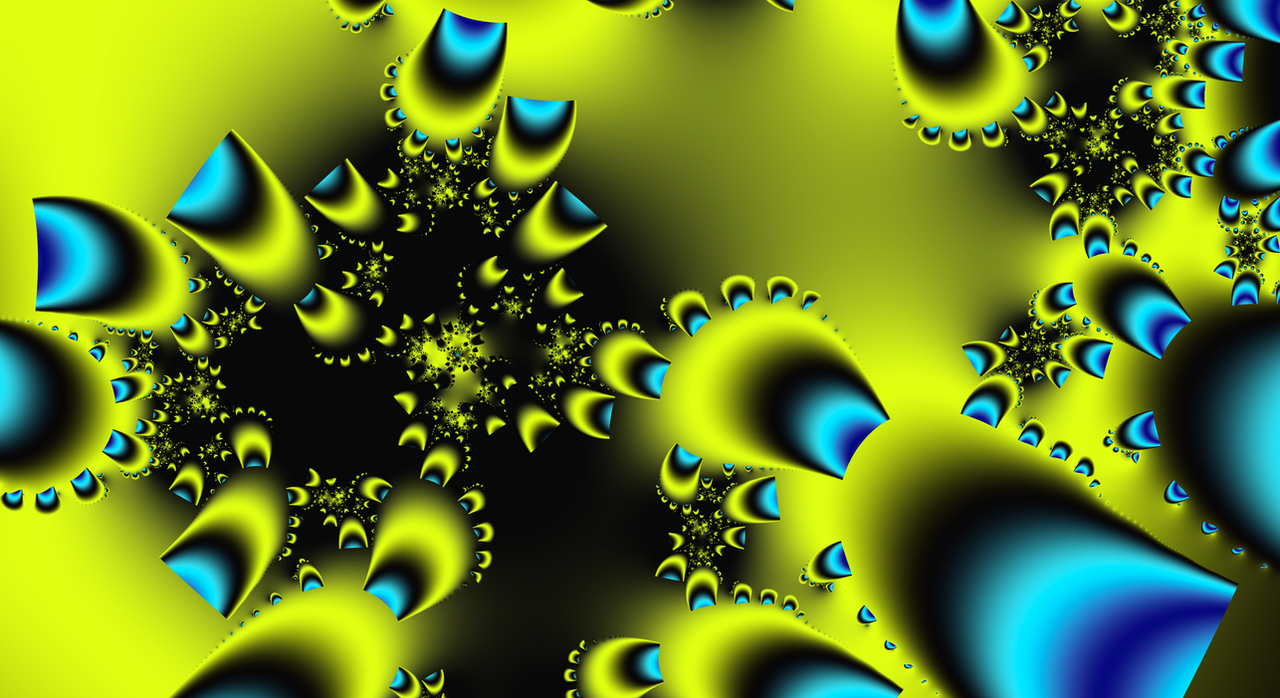
#fractalart #fractview
Published: 2020-03-22 00:02:27 +0000 UTC; Views: 413; Favourites: 6; Downloads: 1
Redirect to original
Description
c^z * exp(-z) + z^2FractView for Android
{"source":["// Default Preset","// This is a good start for all kinds of fractals","// including newton sets, nova fractals and others.","var x int, y int, color int;","","","extern maxdepth int \u003d 120;","","// some further arguments","extern juliaset bool \u003d false;","extern juliapoint cplx \u003d -0.8:0.16;","","// c: coordinates, breakcondition: a function whether we should stop, ","// value: a real variable to return some kind of value","// used in 3d-types for the height.","// returns a quat representing the color","func escapetime(c, breakcondition) {","\tvar i int \u003d 0,","\t\tp cplx \u003d juliapoint if juliaset else c,","\t\tzlast cplx \u003d 0,","\t\tz cplx,","\t\tznext cplx \u003d 0;","","\textern mandelinit expr \u003d \"0\";",""," z \u003d c if juliaset else mandelinit;","","\textern function expr \u003d \"mandelbrot(z, p)\";","","\tvar color quat;","","\twhile {","\t\tznext \u003d function;","\t\tnot breakcondition(i, znext, z, zlast, c, p, color)","\t} do {","\t\t// advance to next values","\t\tzlast \u003d z;","\t\tz \u003d znext;","\t}","","\t// return color","\tcolor","}","","// everything that is drawn must have a get_color-function.","","// c \u003d coordinates (scaled)","// value is a real variable for z-information in 3D","// but also otherwise convenient to separate drawing","// algorithm from transfer","// returns color.","func get_color(c, value) {","","\t// if the fractal accumulates some values","\t// like in traps or addends, here is a got place to do it.","","\tfunc breakcondition(i, znext, z, zlast, c, p, color) {","\t\tfunc bailoutcolor() {","\t\t\textern bailout real \u003d 128;","\t\t\textern max_power real \u003d 2;","\t\t\tvar smooth_i \u003d smoothen(znext, bailout, max_power) ;",""," // the next ones are only used in 3d-fractals","\t\t\textern bailoutvalue expr \u003d \"i + smooth_i\";","\t\t\tvalue \u003d bailoutvalue ;","\t\t","\t\t\textern bailouttransfer expr \u003d \"log(value + 19)\";","","\t\t\textern bailoutpalette palette \u003d [","\t\t\t\t\t[#006, #26c , #fff , #fa0 , #303]];","\t","\t\t\tcolor \u003d bailoutpalette bailouttransfer","\t\t}","","\t\tfunc lakecolor() {","\t\t\textern epsilon real \u003d 1e-9;","\t\t","\t\t\t// the next ones are only used in 3d-fractals","\t\t\textern lakevalue expr \u003d \"rad znext\";","\t\t\tvalue \u003d lakevalue;","","\t\t\textern laketransfer expr \u003d","\t\t\t\t\"arcnorm znext : log(1 + value)\";","","\t\t\textern lakepalette palette \u003d [","\t\t\t\t[#000, #000, #000, #000],","\t\t\t\t[#f00 , #ff0 , #0f8 , #00f ],","\t\t\t\t[#f88 , #ff8 , #afc , #88f ]];","","\t\t\tcolor \u003d lakepalette laketransfer","\t\t}","","\t\t{ lakecolor() ; true } if not next(i, maxdepth) else","\t\tradrange(znext, z, bailout, epsilon, bailoutcolor(), lakecolor())","\t}","\t","\tescapetime(c, breakcondition)","}","","func get_color_test(c, value) {","\t// this one is just here for testing light effects"," // circle + donut + green bg"," var rc \u003d rad c;"," "," { value \u003d (circlefn rc + 5); int2lab #0000ff } if rc \u003c 1 else"," { value \u003d circlefn abs (rc - 3); int2lab #ff0000 } if rc \u003d\u003c 4 and rc \u003e\u003d 2 else"," { value \u003d -10; int2lab #00ff00 }","}","","// ******************************************","// * Next are just drawing procedures. They *","// * should be the same for all drawings. * ","// ******************************************","","extern supersampling bool \u003d false;","extern light bool \u003d false;","","// drawpixel for 2D","func drawpixel_2d(x, y) { ","\tvar c cplx \u003d map(x, y);","\tvar value real;","\tget_color(c, value) // value is not used","}","","// drawpixel for 3D","func drawpixel_3d(x, y) {","\tvar c00 cplx \u003d map(x, y),","\t\tc10 cplx \u003d map(x + 1, y + 0.5),","\t\tc01 cplx \u003d map(x + 0.5, y + 1);","\t","\tvar h00 real, h10 real, h01 real; // heights","\t","\t// color is already kinda super-sampled","\tvar color \u003d (get_color(c00, h00) + get_color(c10, h10) + get_color(c01, h01)) / 3;","","\t// get height out of value","\tfunc height(value) {","\t\textern valuetransfer expr \u003d \"value\";","\t\tvaluetransfer","\t}","\t","\th00 \u003d height h00; h01 \u003d height h01; h10 \u003d height h10;","","\t// get the normal vector (cross product)","\tvar xp \u003d c10 - c00, xz \u003d h10 - h00;","\tvar yp \u003d c01 - c00, yz \u003d h01 - h00;","\t","\tvar np cplx \u003d (xp.y yz - xz yp.y) : (xz yp.x - xp.x yz);","\tvar nz real \u003d xp.x yp.y - xp.y yp.x;","\t\t","\t// normalize np and nz","\tvar nlen \u003d sqrt(rad2 np + sqr nz);","\tnp \u003d np / nlen; nz \u003d nz / nlen;","\t\t","\t// get light direction","\textern lightvector cplx \u003d -0.667 : -0.667; // direction from which the light is coming","\tdef lz \u003d sqrt(1 - sqr re lightvector - sqr im lightvector); // this is inlined","","\t// Lambert\u0027s law.","\tvar cos_a real \u003d dot(lightvector, np) + lz nz;","","\t// diffuse reflexion with ambient factor","\textern lightintensity real \u003d 1;","\textern ambientlight real \u003d 0.5;","","\t// if lumen is negative it is behind, ","\t// but I tweak it a bit for the sake of the looks:","\t// cos_a \u003d -1 (which is super-behind) \u003d\u003d\u003e 0","\t// cos_a \u003d 0 \u003d\u003d\u003e ambientlight","\t// cos_a \u003d 1 \u003d\u003d\u003e lightintensity","","\t// for a mathematically correct look use the following:","\t// if cos_a \u003c 0 then cos_a \u003d 0;","\t// color.a \u003d color.a * (ambientlight + lightintensity lumen);","\t","\tdef d \u003d lightintensity / 2; // will be inlined later","","\t// Change L in Lab-Color","\tcolor.a \u003d color.a (((d - ambientlight) cos_a + d) cos_a + ambientlight);","","\t// Next, spectacular reflection. Viewer is always assumed to be in direction (0,0,1)","\textern spectacularintensity real \u003d 1;","","\textern shininess real \u003d 8;","","\t// r \u003d 2 n l - l; v \u003d 0:0:1","\tvar spec_refl \u003d 2 cos_a nz - lz;","\t","\t// 100 because L in the Lab-Model is between 0 and 100","\tif spec_refl \u003e 0 then","\t\tcolor.a \u003d color.a + 100 * spectacularintensity * spec_refl ^ shininess;","","\tcolor","}","","func do_pixel(x, y) {","\t// two or three dimensions?","\tdef drawpixel \u003d drawpixel_3d if light else drawpixel_2d;","\t","\tfunc drawaapixel(x, y) {","\t\t0.25 (","\t\t\tdrawpixel(x - 0.375, y - 0.125) + ","\t\t\tdrawpixel(x + 0.125, y - 0.375) + ","\t\t\tdrawpixel(x + 0.375, y + 0.125) +","\t\t\tdrawpixel(x - 0.125, y + 0.375)\t\t\t","\t\t);","\t}","","\t// which function to apply?","\tdef fn \u003d drawpixel if not supersampling else drawaapixel;","","\tcolor \u003d lab2int fn(x, y)","}","","// and finally call the draing procedure","do_pixel(x, y)"],"arguments":{"ints":{"maxdepth":5800},"exprs":{"function":"p^z * exp(-z) + z^2","bailouttransfer":"log(value / p - value^p)"},"palettes":{"bailoutpalette":{"width":9,"height":1,"colors":[-16777216,-2097390,-16777216,-16717825,-16250496,-16717825,-16777216,-2097390,-16777216]}},"scales":{"Scale":[-0.0018135622361079152,-1.770036769818404E-4,1.770036769818404E-4,-0.0018135622361079152,-2.7863410338969827,-1.3361792362558156]}}}